CS 2120: Topic 4¶
Videos for this week:¶
Conditionals¶
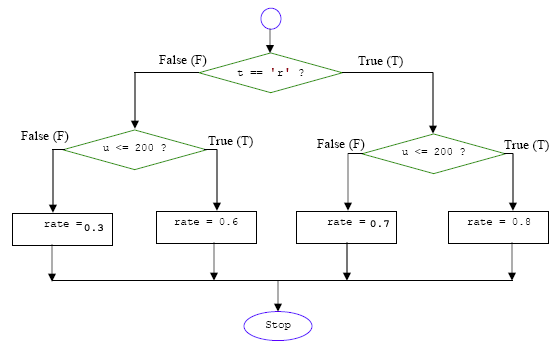
Try this..
Using only the Python statements, try to write a program that will divide a number in half only if that number is a multiple of 2.
Conditionals (or conditional execution) will make these types of tasks easier.
Logic¶
To make parts of the program conditionally executed, we need a formal way to describe conditions.
We need logic.
- Let’s try some comparison:
>>> 19 == 87 False >>> 5==5 True
Note that
==
is comparison while=
is assignment. They are not the same.
Try this..
Figure out what the other comparison operators in Python are. Hint: 3
doesn’t equal 5
.
- These operators can be applied to any two expressions (could be simply a value or variable, but can be more complex):
>>> a=15 >>> b=37 >>> (a+b)*9 > (b-a)*3 + 2 True
Question
What is the type of the result of applying a comparison operator?
Conditional execution¶
Comparison statements can be
True
orFalse
, but how do we use this to control our program?if
some condition isTrue
, do something:if grade < 50: print('Ya I don't think I passed..')
If the condition following the keyword
if
isTrue
, the block of code after the:
gets executed.If the condition is
False
, the block inside gets skipped over.The blocks can be as long as you want (but the minimum size is 1)
Try this..
Using only the Python features/statements we’ve seen so far, can you write a program that will divide a number in half only if that number is a multiple of 2?
Compound conditions¶
We can use the logical operators
and
,or
andnot
to combine conditions:if (Bob_has_cineplex_membership == True and Is_Friday == True) or (Bob_complains): print('Bob gets to go into the movie for free.')
For more on logical operators..
Look at the truth tables for the logical operations AND, OR and NOT for help here.Wikipedia link: Wikipedia link.
Alternative execution¶
if
statements can have a specialelse
statement to go with them:if x > 10: do_something() else: do_something_else()
Chaining alternative execution¶
Sometimes just having
if
/else
isn’t enough.What if I want several, mutually exclusive, alternatives?:
if year < 1960: print('Jazz') elif year < 1980: print('Rock') elif year < 1990: print('Synthpop') elif year < 2003: print('Alternative') elif year < 2012: print('Hip Hop') else: print("The Lils")
elif
meanselse if
NOTE: Once one of the
elifs
gets executed, that’s it. The remaining ones are ignored.You can have as many elifs as you want
Always end with a plain
else
as a “catch-all”.
Nested conditionals¶
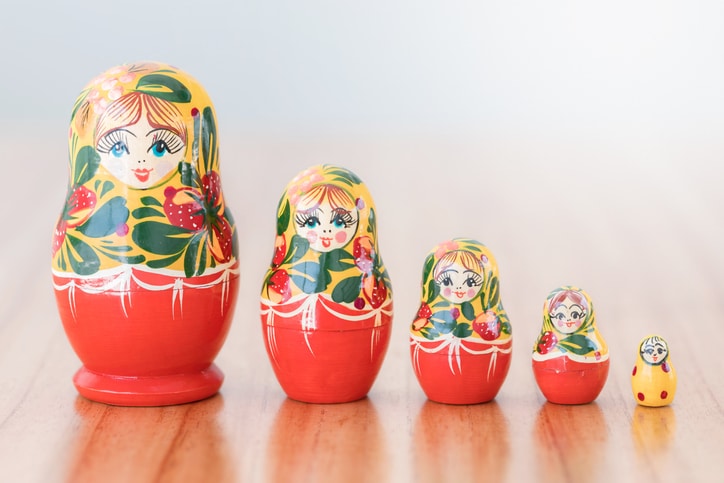
It’s also possible to nest conditionals inside other conditionals:
if x > 0: if y > 0: print('First Quadrant') else: print('Fourth Quadrant') else: if y > 0: print('Second Quadrant') else print('Third Quadrant')
There’s no limit to how deep you can nest.
Libraries¶
Python has a huge variety of existing modules and libraries/packages.
Modules are just files which contains python code which you can import for use in, for example, a python script. Modules have definitions (i.e. function definitions) and statements
Packages are collections of modules
Libraries are a collection of modules and/or packages.
The Python docs have more on this (here)
No matter what you want to do, there’s probably a library that can help you. We’ve already used pandas in assignment 1. Let’s look at NumPy
NumPy¶
Numerical Python or “NumPy”
included with Anaconda
- Like pandas, though, it isn’t ‘built in’ to Python, so we have to tell the interpreter that we want to use NumPy::
>>> import numpy
NumPy Types¶
Recall that Python values have types.
NumPy has its own types.
- We can specify these::
>>> x = numpy.float32(7.3) >>> print(x) 7.3 >>> type(x) <type 'numpy.float32'>
You can convert between regular Python types and NumPy types.