CS 2120: Topic 5¶
Videos for this week:¶
Extra Video:¶
Filling in the gaps¶
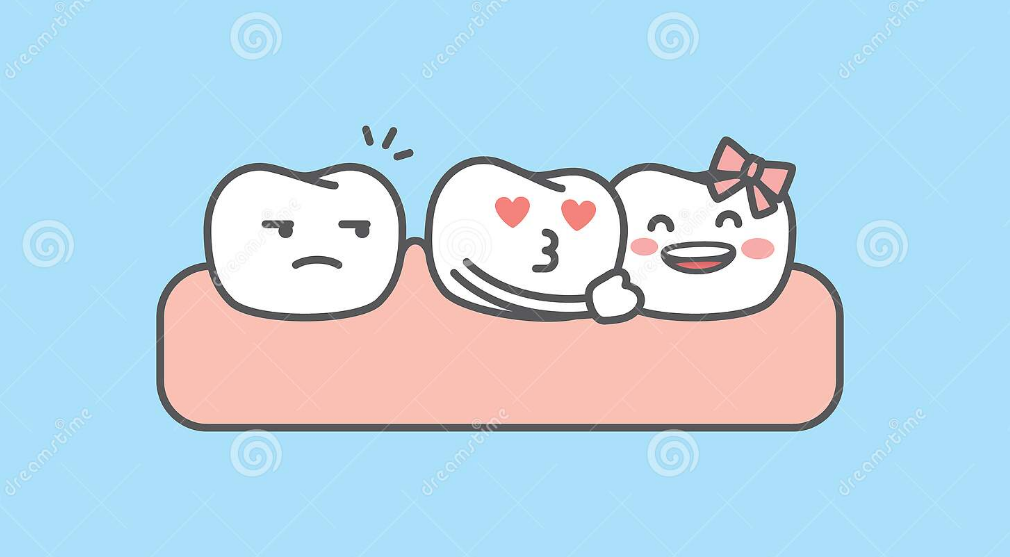
More about booleans¶
We’ve used comparison operators (e.g.,
<,>,==
) in conditionals.- What’s going on “under the hood” with the comparison, though?
>>> 5>2 True >>> 5<2 False
A comparison like
a>b
is just an expression, likea+b
.. but the value it produces isn’t an integer, it’s eitherTrue
orFalse
- A value that is either
True
orFalse
(and nothing else) has type *Boolean*: >>> type(5>2) <type 'bool'>
- A value that is either
Try this..
Write a function
is_negative(n)
that returnsTrue
if the argumentn
is negative andFalse
otherwise.
More about returns¶
We’ve seen functions that print, and functions that return a value (or list) at the end. What else can we do?
Lots.. but here’s another one.. we can have multiple returns:
def divisible_by(a,b): if a % b == 0: return True else: return False
Try this..
What is the result of the function call divisible_by(4,2)
? How about divisible_by(4,3)
? Write a new
function not_divisible_by(a,b)
that returns True
when a
is not divisible by b
and False
otherwise.
The function type¶
- In Python, functions have a type, too:
>>> type(divisible_by) <type 'function'>
You can check out first-class functions, but you don’t have to.. it’s a bit silly.
**The point**
: This allows us to quickly write code that is very generic:def add(a,b): return a+b def subtract(a,b): return a-b def dosomething(f,a,b): return f(a,b)
Try this..
What is the value of dosomething(add,5,7)
? How about dosomething(substract,5,7)
Writing larger programs¶
Many larger python programs are made up of hundreds (or thousands) of lines with multiple functions. In constructing these larger programs, here’s some general advice (which you may find useful):
Break your overall problem into a heirarchy of subproblems
i.e. “Write a calculator program” -> you’ll need an “add” function, a “subtract” function, …
When creating a particular function..
define it and set it to return a constant value (i.e. 0):
def colourOfPixel(image,x,y): return 0
does it work? Ok, next try to add a few lines of code to accomplish some of what you want to do:
def colourOfPixel(image,x,y): image = Image.open(image) image_rgb = image.convert('RGB') # rest of code will go below
does it work? Ok, continue to build incrementally and test at each increment.
Once you’ve written of your low level functions, start combining to write the higher level ones.
This chunking
of a larger problem into subproblems is very important for coding. Yes.. you could just type it all out in one shot, but as your programs get larger this will become more difficult (and will take more time).
For next class¶
Complete Activity 1 and submit it on OWL by the end of the week (Friday October 09 @ 11:59 PM)