CS 2120: Topic 6¶
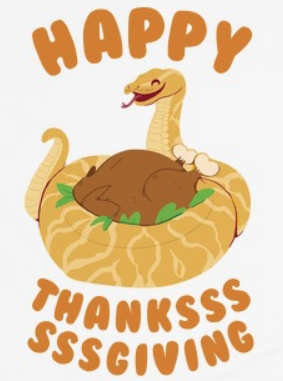
Videos for this week:¶
Correction
@ 6:40 in video 2, the return
statement is not needed.
Reusing variables¶
Try this..
Consider this code:
a = 5
print(a)
b = 6
print(a)
a = a+b
print(a)
a = 3
a = a+1
print(a)
What is the value of the variable a
at each of the print statements?
The point: we can reuse the same variable, reassigning it to a new value:
a = a + 1
The while loop¶
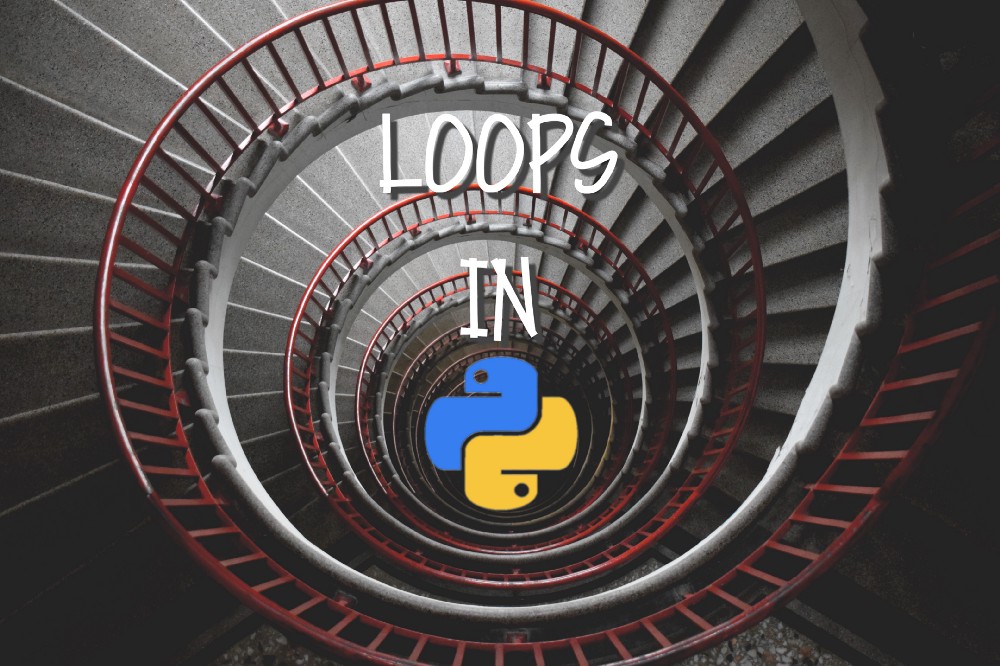
So far, if we want Python to do the same thing over and over, we have to tell it explicitly by repeating those instructions over and over.
Question: How do we automate this?
Answer: the
while
statement.
The while statement
“While some condition is true, keep doing the code in the indented block”:
a = 1
while a < 11:
print(a)
a = a + 1
What is happening here?
Before the
while
statement, we initialize the loop variablea
The
while
statement is followed by a condition (any Boolean expression).. if the condition isTrue
, the body of the loop gets executed, otherwise it gets skipped.
Question
What would happen if we didn’t have a=a+1
?
Consider this code:
def dostuff(n): answer = 1 while n > 1: answer = answer * n n = n - 1 return answer
Tracing through code
What does the code above do? Trace through it, using pen and paper, for a few example values of n
.
To trace, we can build a table of values.
Let’s trace
dostuff(4)
. We’ll look at the values ofn
andanswer
right after thewhile
statement.
n |
answer |
---|---|
4 |
1 |
3 |
4 |
2 |
12 |
Side note: In Python, the pattern
a = a + 1
can be written asa += 1
.
Encapsulation¶
In the scope of the course, when we refer to
encapsulation
we are talking about putting some piece of code into a function.
Generalization¶
In the scope of the course, when we refer to
generalization
, we are talking about making something more general (i.e.n
instead of5
)
Example of Encapsulation
Consider this while loop:
while(i<5):
print(2*i)
i = i + 1
We can encapsulate it like this:
def print_multiples():
while(i<5):
print(2*i)
i = i + 1
Example of Generalization
Let’s generalize the previous function:
def print_multiples(n):
while(i<5):
print(n*i)
i = i + 1
For next class¶
Complete Activity 2 and submit it on OWL by the end of the week (Friday October 16 @ 11:59 PM)