CS 2120: Topic 7¶
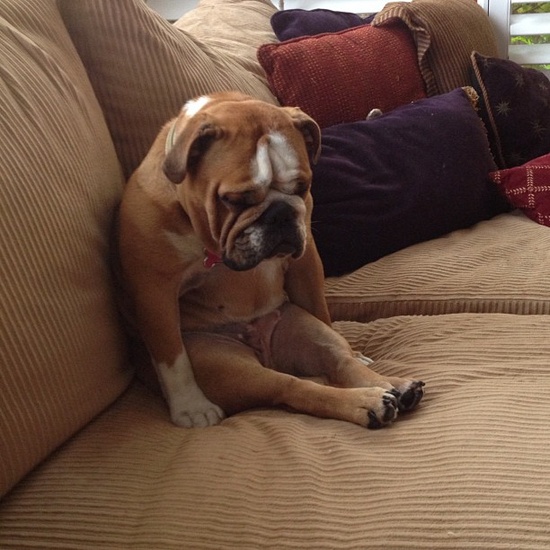
Videos for this week:¶
A note on comments¶
Helpful for others who are reading your code (they make it easier to read and understand)
Helpful for you when you revisit your own code (i.e. when you return to a project a month later)
You can add comments to your code in Python with
#
:# we can comment above my_car = 5 #or to the right
Once Python sees
#
it ignores the rest of the current lineDon’t just repeat what’s obvious from reading the code… provide a higher level description of what’s happening.
Python docstrings¶
These are comments for functions (also other things like methods, classes, etc.)
Used immediately after the function definition:
def set_up_cities(names): """ Set up a collection of cities (world) for our simulator. Each city is a 3 element list, and our world will be a list of cities. :param names: A list with the names of the cities in the world. :return: a list of cities """ function code here
Explain what the function is going to do, in plain English.
Also explain every parameter and what the function returns
More on strings¶
Question: How is a string different from the other data types we’ve seen (
int
,float
,bool
, etc.)?Answer: We can print individual characters of a string by indexing the string:
>>> a='Boomboombobo' >>> print(a[0]) B >>> print(a[1]) o
Note that in Python, the first index is
0
, not1
What does
print(a[0:4])
do?>>> print(a[0:4]) Boom
This process is called slicing.
More on loops¶
while
loops are great, but they rely on a boolean condition returning false (i.e. while condition is true, do something):while(mouse_not_yet_clicked = True): print("Please click the mouse")
for
loops allow for iteration through a specified range or over elements in a list (among others):# one way you may use a for loop for i in range(len(a)): print(a[i])
for i from 0 until the length of the string a, print the ith element of a:
# another way you may use a for loop for char in a: print(char)
for each character in the string
a
, we run the indented code block.Yes, you could create a
while
loop which does exactly the same thing… thefor
loop is just cleaner here (less typing).
Mutability¶
We can access an individual character in a string with an index (i.e. a[0])… can we set an individual character by index as well?
Let’s try:
>>> a[7]='x' Traceback (most recent call last): line 4, in <module> a[7] = 'x' TypeError: 'str' object does not support item assignment
Variables of some types are mutable… i.e. they can be changed.
Strings are immutable — you can’t change a string, you have to make a new one:
>>> new_a = a[:7] + 'x' + a[8:] >>> print(new_a) Boombooxbobo
Python Lists¶
You’ve seen these in Assignment 1, and maybe you made your own in Activity 2
What is it? An ordered and changeable collection of values or elements
Lists are like strings, except that the elements can be any type (not just characters):
>>> myList = []
>>> myList.append(5)
>>> print(myList)
[5]
>>> myList.append(10)
>>> print(myList)
[5,10]
>>> print(myList[0])
5
We can add to the end of lists with
append
Like strings, we can access the elements of a list with myList[i], where
i
is the list’s index (starts from 0 for the first element)
For next class¶
Take a look at Assignment 2 (due October 30th)