CS 2120: Topic 9¶
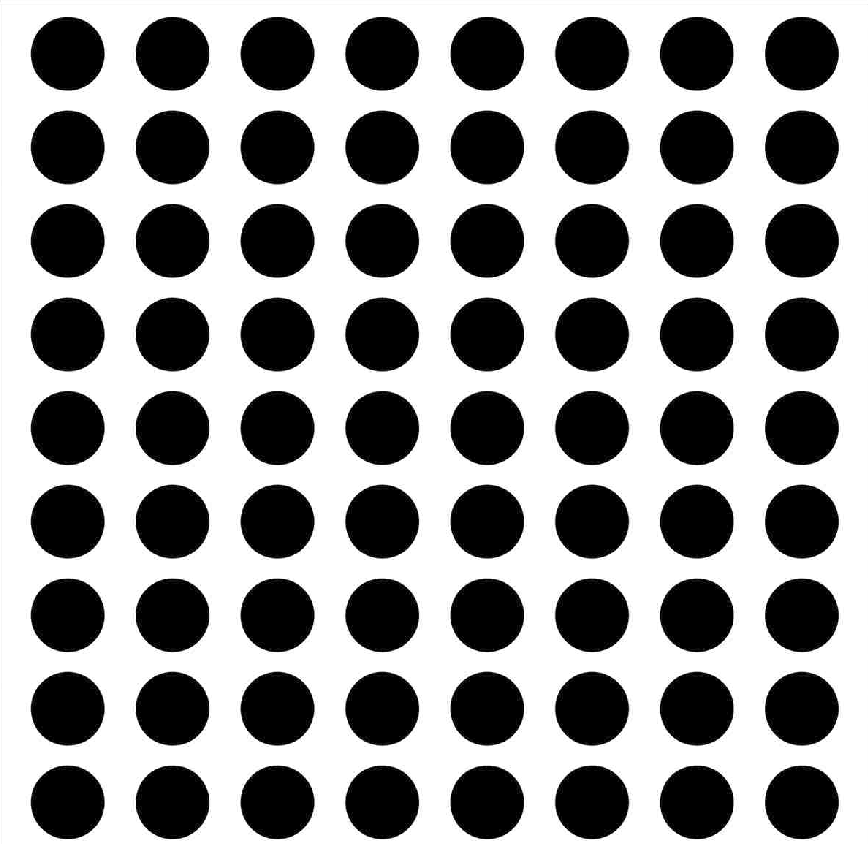
Videos for this week:¶
Numpy arrays¶
Another data structure… the array:
>>> a=numpy.array([5,4,2]) >>> print(a) [5 4 2]
We can access elements of the array (like we do with a list):
>>> print(a[0]) 5 >>> print(a[1]) 4
Like lists, arrays are also mutable:
>>> a[1]=7 >>> print(a) [5 7 2]
To check the type of a numpy array’s elements
>>> a.dtype dtype('int64')
Notice that usually we’d type this to get the type of something…
>>> type(something)
So, here we are instead asking NumPy to give us the data type (
dtype
) attribute of our arrayTo see all of the attributes your array has:
>>> dir(a)
i.e. to change the type of an array’s elements:
>>> b=a.astype(numpy.float32) >>> b.view() array([ 5., 4., 2.], dtype=float32)
You can’t technically “append” to an array, but you can create a new array with added values using
numpy.append()
:>>> a = numpy.array([1,2,3,4]) >>> a.view() array([1, 2, 3, 4]) >>> b = numpy.append(a,5) >>> a.view() array([1, 2, 3, 4]) >>> b.view() array([1, 2, 3, 4, 5])
Note:
numpy.append()
did not change a. It created a new array, b.
Higher Dimensional Arrays¶
Let’s create a 2D array:
>>> a=numpy.array([[1,2,3],[4,5,6],[7,8,9]]) >>> a.view() array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
As long as two 2D arrays have the same shape, you can do arithmetic on them, just like 1D arrays.
>>> a.shape (3, 3)
If you want an array of zeros, of shape
(n,m)
:>>> a=numpy.zeros([n,m])
Also try
numpy.ones()
andnumpy.eye()
We can slice 1D, 2D, or higher dimensional arrays:
>>> a=numpy.arange(25).reshape(5,5) >>> a.view() array([[ 0, 1, 2, 3, 4], [ 5, 6, 7, 8, 9], [10, 11, 12, 13, 14], [15, 16, 17, 18, 19], [20, 21, 22, 23, 24]]) >>> print(a[0:2,1:4]) [[1 2 3] [6 7 8]]
Here,
numpy.arange
works likerange
but returns an array.If you use
:
as an index you can get an entire row or column:>>> print(a[:,0:2]) [[ 0 1] [ 5 6] [10 11] [15 16] [20 21]]
Further reading
There’s a LOT more that NumPy can do… if you’re interested, take a look at the NumPy Quickstart Tutorial for more info.
Tuples¶
A tuple looks a lot like a list, but with
()
instead of[]
:>>> tup = (3,5) >>> print(tup) (3, 5)
What is the point of tuples?
Anything you can do with tuples, you can do with lists. But…
tuples are immutable
they are more compact than lists and therefore faster (but you won’t notice this until you have many elements)
Dictionaries¶
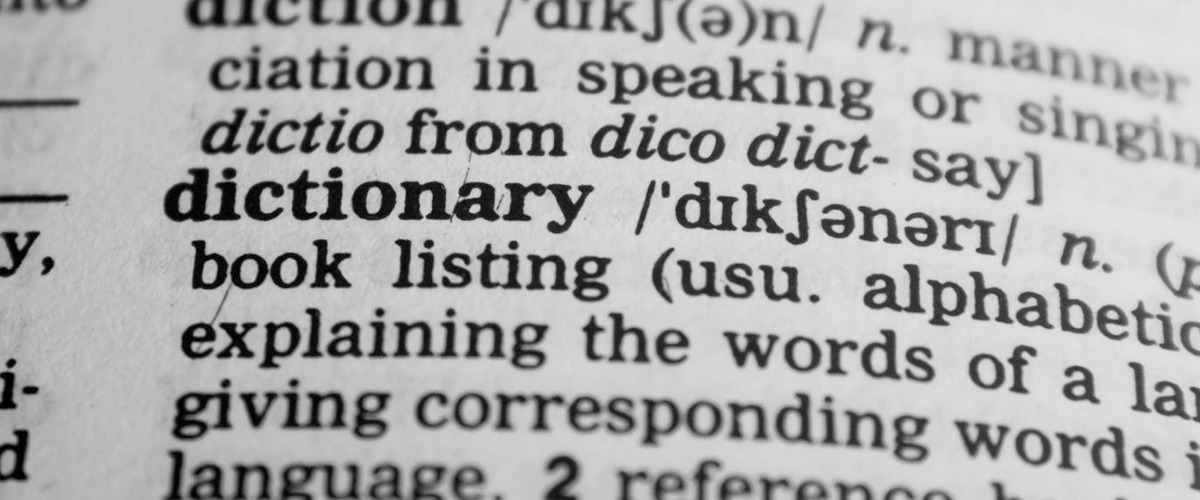
Along with lists, arguably one of the most important Python data structures
These are basically lists which can be indexed with strings instead of numbers.
Another way of thinking of them — a set of
key-value pairs
Let’s create an empty dictionary:
>>> mydict = {}
Let’s add to it…
>>> mydict['Simon']=50 >>> print(mydict) {'Simon': 50}
The key
Simon
is associated with the value50
.Let’s add more…
>>> mydict['Suzy'] = 95 >>> mydict['Johnny'] = 85 >>> print(mydict) {'Suzy': 95, 'Johnny': 85, 'Simon': 50}
The key
Simon
is associated with the value50
.Dictionaries always associate a key with a value
i.e.
dict[key] = value
Dictionaries are a generalization of lists
A list associates fixed indices from 0 up to
n
with values.A dictionary associates arbitrary strings with values.
Before we finish, try the following commands:
>>> mydict.keys() >>> mydict.values()
For next class¶
Your final activities and assignments for the course are available or will be released this week
Activity 3 is out (and optional), Assignment 3 will be out in the next few days, and Assignment 4 will be released shortly after (near the end of the week)… please take a look at each of these as they are released.
- Deadlines:
Activity 3 — Nov. 13 @ 11:59 pm
Assignment 3 — Nov 20 @ 11:59 pm
Assignment 4 — Dec 04 @ 11:59 pm