Exam Practice¶
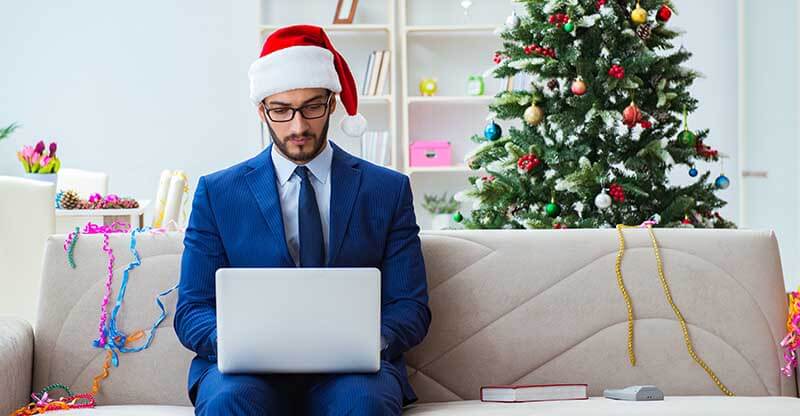
Videos (reviewing this page)¶
Some practice questions…¶
On this page you’ll find a sampling of practice questions for the exam. Note that these will be taken up at the end of the week — I will post a corresponding video.
The exam will be broken into 3 categories:
Multiple Choice
True/False
Short Answer
In terms of content, all weeks are included, but I am mostly looking to fill the exam with material from Weeks 1 - 10 — questions from Week 11 would be surface-level (again, think more along the lines of “What’s the difference between supervised and unsupervised machine learning?” and less along the lines of “code an SVM from scratch”).
For Week 10…
Addressing the comments I made on the Week 10 (Recursion) video, recursion will be included on the exam as a possible topic to draw a question/questions from. Question 9 on this page is an example. Another thing to note for week 10: You will not have to memorize any of the algorithms
.
Next week, I will release a practice-exam
: a 1-hour exam which you can complete (optional) in order to prepare for the full exam. For now, I will set it up as a timed
exam (let’s say next Monday from 1 PM - 2 PM) via Proctortrack, but I will make it available afterwards for you to practice with until the exam date.
Below you will find some sample questions from each category, to give you an idea of the “flavour” of the types of questions you could be asked.
Multiple Choice¶
Question 1
Consider this code:
>>> list1 = ['a', 'b', 'c', 'd']
>>> list2 = []
>>> for i in range(len(list1)):
list2.append(list1)
>>> print(list2)
What does this code do?
append list1 to list2
append list2 to list 1
append 4 lists (each a “copy” of list1) to list2
append 3 lists (each a “copy” of list1) to list2
Question 2
Consider this pseudocode:
Create empty list called "list1"
Create empty list called "list2"
For i from i = 0 to (and including) 9:
Append random number to list1
Append random number to list2
j = 0
while(length of list1 < (2 * length of list2)):
Append list2[j] to list1
increment j by 1
How many elements are in list1?
It’s not possible to tell, as it’s a random number
19
20
10
Question 3
What is a “docstring”?
A document which pandas uses to parse csv files.
A string formed from a Microsoft Word “docx” file
A multi-line comment used after defining a function
A “documented” string with
param names
andreturn
. It returns a list of cities.
True/False¶
Question 4
=
is the same as ==
in Python
True
False
Justify your answer.
Question 5
The following code creates an empty dictionary called “my_dict”:
>>> my_dict = {None: None}
True
False
Justify your answer.
Question 6
A function may have multiple arguments.
True
False
Short Answer¶
Question 7
Consider the following Python code:
>>> my_value = 10
>>> if my_value > 15:
>>> print("Cats are the best!")
>>> my_value += 6
Does the print statement get printed? Why/Why not?
Question 8
Write a Python program which uses a loop to print values from 1 to 100, skipping each number which is divisible by 3.
Question 9
Using recursion, define and implement a function called “sum_from_1_to_n(num)” which takes an integer num
as its argument and returns the sum of all numbers from 1 to (and including) n.
i.e. sum_from_1_to_n(3)
would return 6
, which is 3 + 2 + 1.